Home>How-To Guides>Troubleshooting>Troubleshooting Android Confirm Password Issue
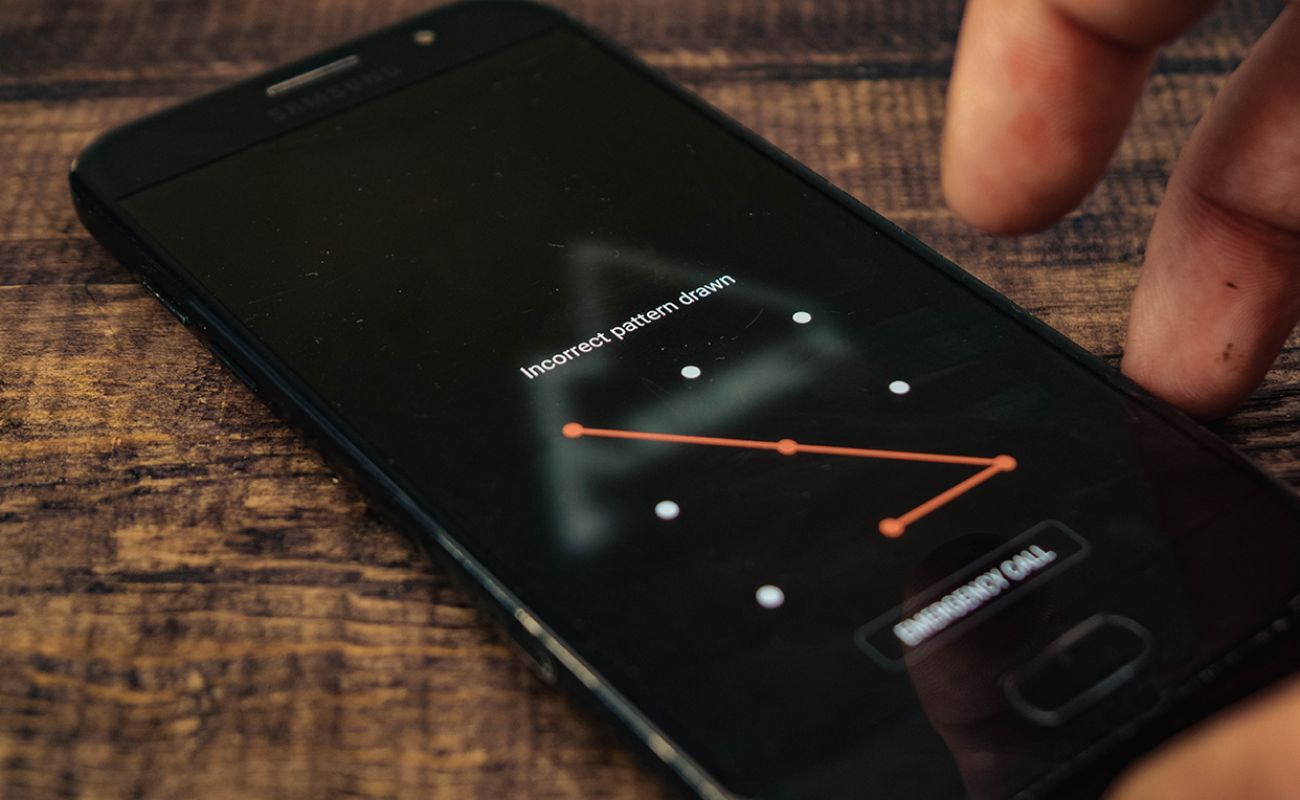
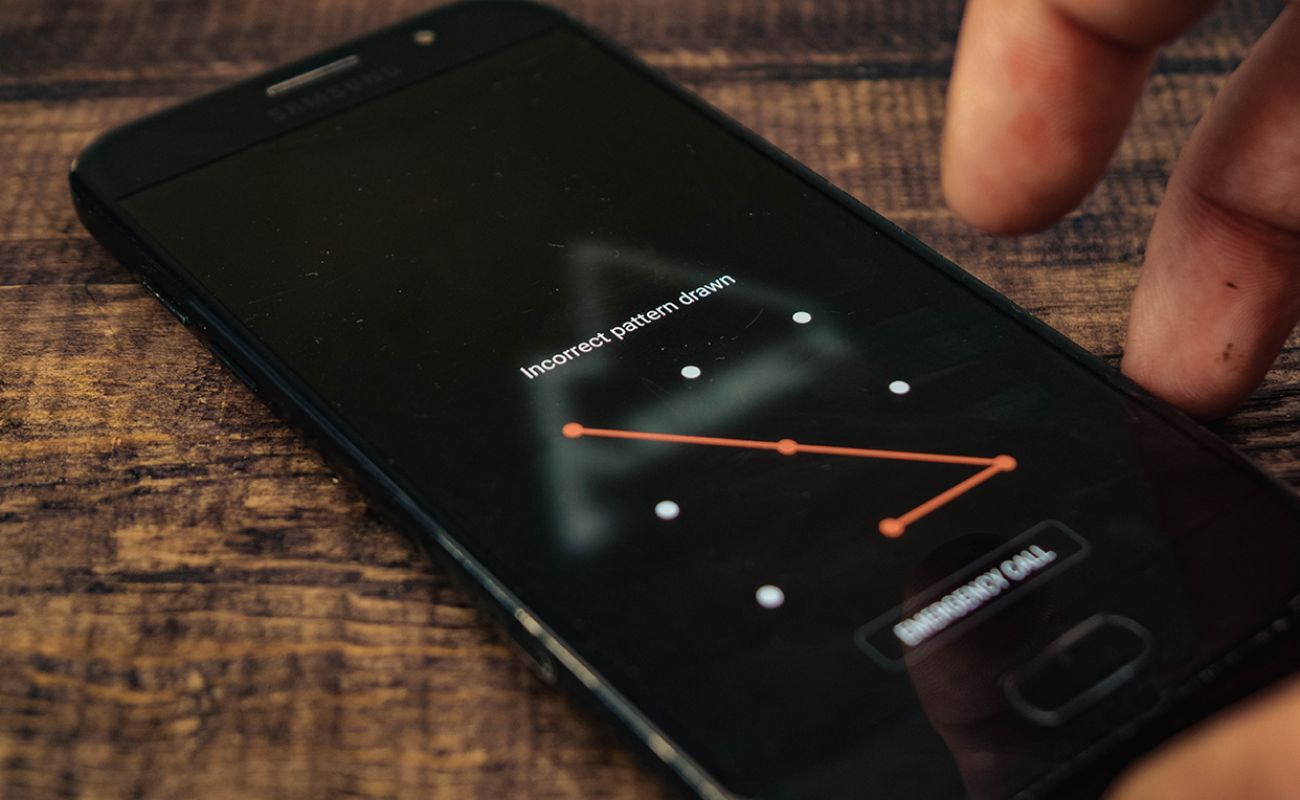
Troubleshooting
Troubleshooting Android Confirm Password Issue
Published: March 4, 2024
Need help troubleshooting the Android confirm password issue? Find solutions and tips to resolve this problem and secure your device.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for Techsplurge.com, at no extra cost. Learn more)
Table of Contents
Common Causes of Android Confirm Password Issue
-
Typing Error: One of the most common causes of the Android confirm password issue is a simple typing error. Users may inadvertently mistype their password, leading to a mismatch during the confirmation process.
-
Password Complexity: Android devices often require users to create complex passwords with a combination of letters, numbers, and special characters. If the user forgets the exact combination or sequence, it can result in a failed password confirmation.
-
Forgotten Password: Human memory is fallible, and it's not uncommon for users to forget their passwords, especially if they haven't used their device for an extended period.
-
Outdated Software: In some cases, outdated software on the Android device can lead to password confirmation issues. Compatibility problems between the operating system and the password confirmation mechanism may arise, causing the process to fail.
-
Biometric Authentication Failure: Many Android devices offer biometric authentication options such as fingerprint or facial recognition. If these features malfunction or encounter errors, users may be prompted to confirm their password as an alternative, leading to potential issues.
-
System Glitches: Occasionally, system glitches or software bugs within the Android operating system can disrupt the password confirmation process, resulting in an inability to confirm the password successfully.
-
Security Settings: Overly stringent security settings or third-party security applications may interfere with the password confirmation process, causing unexpected errors.
Understanding these common causes of the Android confirm password issue is crucial for effectively troubleshooting and resolving the issue. By identifying the root cause, users can take appropriate steps to rectify the problem and regain access to their Android device.
How to Reset Password on Android Device
Resetting a password on an Android device is a straightforward process that can be accomplished through various methods, offering users the flexibility to regain access to their devices in the event of a forgotten or malfunctioning password. Here are the steps to reset a password on an Android device:
1. Utilize Google Account Credentials
If a user has associated their Android device with a Google account, they can leverage this integration to reset their password. By entering the incorrect password multiple times, the device will prompt the user to sign in using their Google account credentials. Upon successful authentication, users can reset their device's password, granting them access to their device.
2. Factory Reset
In situations where a user is unable to access their device due to a forgotten password, a factory reset can be performed to restore the device to its original settings. This process erases all data and settings on the device, including the password, effectively allowing the user to set up a new password upon reinitializing the device.
3. Utilize Android Device Manager
Android Device Manager, now known as Find My Device, offers a feature that enables users to remotely lock their device and set a new password. By accessing the Find My Device website or app from another device, users can initiate the password reset process, providing a seamless solution for regaining access to their Android device.
4. Use Third-Party Recovery Tools
In cases where traditional methods are ineffective, users can explore third-party recovery tools designed specifically for Android devices. These tools often provide advanced features for bypassing or resetting passwords, offering an alternative solution for users encountering password-related issues.
By following these methods, users can effectively reset their passwords on Android devices, ensuring seamless access to their devices and minimizing disruptions caused by forgotten or malfunctioning passwords. It is important to note that while these methods offer viable solutions, users should exercise caution and ensure the security of their data throughout the password reset process.
Troubleshooting Android Confirm Password Issue with Biometric Authentication
Biometric authentication, including fingerprint and facial recognition, has become a popular and convenient method for unlocking Android devices. However, users may encounter issues with biometric authentication, leading to the need to confirm their password as an alternative. Troubleshooting the Android confirm password issue in relation to biometric authentication involves several steps to identify and resolve potential issues.
1. Clean Biometric Sensors
Over time, biometric sensors on Android devices can accumulate dirt, oil, or residue, impacting their ability to accurately recognize fingerprints or facial features. Cleaning the biometric sensors with a soft, lint-free cloth can help improve their functionality and reduce authentication errors, potentially resolving the need for password confirmation.
2. Re-Enroll Biometric Data
In some cases, re-enrolling biometric data can address authentication issues. Users can access the device settings to delete existing biometric profiles and re-register their fingerprints or facial features. This process ensures that the biometric data is accurately captured and stored, potentially resolving inconsistencies that lead to password confirmation prompts.
3. Update Biometric Authentication Software
Ensuring that the device's biometric authentication software is up to date is crucial for addressing potential compatibility issues and security vulnerabilities. Users should check for software updates through the device settings or the manufacturer's official website to install the latest biometric authentication software, which may contain bug fixes and enhancements to improve authentication accuracy.
4. Adjust Biometric Settings
Android devices offer various settings related to biometric authentication, including sensitivity adjustments and additional security measures. Users can explore these settings to fine-tune the biometric authentication process, potentially mitigating issues that trigger the need for password confirmation. Adjusting sensitivity levels and security settings can enhance the overall reliability of biometric authentication.
5. Contact Customer Support
If troubleshooting steps fail to resolve the Android confirm password issue related to biometric authentication, users can reach out to the device manufacturer's customer support for further assistance. Customer support representatives can provide personalized guidance, troubleshoot specific device issues, and offer potential solutions tailored to the user's unique circumstances.
By following these troubleshooting steps, users can address the Android confirm password issue associated with biometric authentication, ensuring a seamless and reliable unlocking experience on their Android devices. Identifying and resolving biometric authentication issues contributes to a more secure and user-friendly device usage, enhancing the overall satisfaction and convenience of biometric unlocking methods.
Resolving Android Confirm Password Issue with Software Updates
Software updates play a pivotal role in maintaining the functionality and security of Android devices. When users encounter the Android confirm password issue, leveraging software updates can often serve as a viable solution to address underlying issues and enhance the overall performance of the device.
Importance of Software Updates
Software updates, including operating system upgrades and security patches, are designed to address known vulnerabilities, improve system stability, and introduce new features. By regularly updating their devices, users can benefit from bug fixes, security enhancements, and optimizations that contribute to a seamless and secure user experience.
Addressing Compatibility Issues
In some instances, the Android confirm password issue may stem from compatibility issues between the device's operating system and the password confirmation mechanism. Software updates often include compatibility fixes that address such issues, ensuring that the password confirmation process functions as intended without encountering errors or inconsistencies.
Bug Fixes and Performance Enhancements
Software updates frequently incorporate bug fixes that target specific issues related to password confirmation and overall system functionality. By resolving underlying software bugs, users can experience improved reliability and consistency when confirming their passwords, mitigating the occurrence of unexpected errors or authentication failures.
Security Enhancements
Security is a paramount concern for Android users, and software updates are instrumental in fortifying the device's defenses against potential threats. By staying up to date with software updates, users can benefit from the latest security patches and protocols, reducing the likelihood of security-related issues that may impact the password confirmation process.
Optimizing Biometric Authentication
For devices equipped with biometric authentication features, software updates often include optimizations and refinements to enhance the accuracy and reliability of fingerprint and facial recognition systems. These improvements contribute to a smoother and more seamless biometric authentication experience, reducing the reliance on password confirmation as an alternative unlocking method.
Ensuring Data Privacy and Protection
Software updates also play a crucial role in safeguarding user data and privacy. By addressing potential vulnerabilities and implementing privacy-focused enhancements, updates contribute to a more secure environment for password confirmation processes, instilling confidence in the protection of sensitive information.
By prioritizing software updates and ensuring that their Android devices are running the latest software versions, users can effectively resolve the Android confirm password issue while benefiting from a range of performance, security, and usability improvements. Regularly checking for and installing software updates is a proactive approach that fosters a reliable and secure device usage experience, ultimately enhancing user satisfaction and peace of mind.
Contacting Customer Support for Android Confirm Password Issue
When all other troubleshooting methods have been exhausted and the Android confirm password issue persists, contacting customer support can provide valuable assistance and personalized guidance. Customer support channels offered by the device manufacturer or service provider serve as a direct resource for users to seek resolution to complex technical issues, including password confirmation challenges.
Expert Guidance and Troubleshooting
Customer support representatives possess in-depth knowledge of the device's functionality and can offer expert guidance tailored to the specific circumstances of the Android confirm password issue. By reaching out to customer support, users can articulate their concerns and provide relevant details, enabling the representatives to initiate targeted troubleshooting steps and identify potential root causes of the issue.
Personalized Solutions and Recommendations
Customer support interactions often result in personalized solutions and recommendations based on the user's device model, software version, and unique usage patterns. Representatives may suggest specific actions, such as performing advanced system diagnostics, accessing hidden device settings, or initiating remote troubleshooting procedures to address the password confirmation issue effectively.
Escalation to Technical Specialists
In cases where the Android confirm password issue presents complex or persistent challenges, customer support can escalate the matter to technical specialists or engineering teams within the organization. These specialized teams possess advanced expertise and resources to delve into intricate technical issues, conduct in-depth analysis, and develop targeted solutions to resolve the password confirmation issue.
Firmware or Software Updates
Customer support can provide insights into upcoming firmware or software updates that may contain fixes or enhancements directly related to the password confirmation process. By staying informed about potential updates, users can anticipate improvements that address the specific issue they are encountering, ensuring that their devices remain up to date and optimized for reliable password confirmation.
Warranty and Service Options
In situations where the Android confirm password issue is attributed to hardware or system defects, customer support can guide users through warranty claims and service options. This may involve facilitating device inspections, repairs, or replacements to address underlying issues that contribute to the password confirmation challenges, ensuring that users can regain seamless access to their devices.
By leveraging the expertise and resources offered through customer support channels, users can navigate the complexities of the Android confirm password issue with confidence, knowing that they have access to dedicated assistance and tailored solutions. Customer support interactions serve as a valuable avenue for resolving technical hurdles, empowering users to overcome password confirmation issues and optimize their overall device usage experience.